Push Notification Mobile SDKs
Syniverse Mobile SDKs enable easy integration between the mobile applications and the Syniverse Omni-channel API to send push notifications. The customer have the choice to integrate directly with the APIs but can optionally choose to use the mobile SDKs for integration to simplify the integration within the mobile application.
Syniverse iOS Mobile SDK for Push Notifications.
The SDK is available via CoCoaPods.org
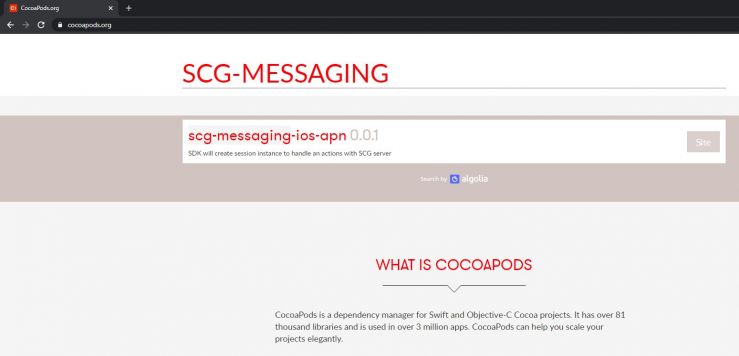
How to integrate the SDK within the mobile application
Step 1 : Before using scg-messaging-ios cocoapod a developer need to install pod utility on target OS with next command.
brew install pod
With pod utility installed, the developer can add the SCG messagin-ios SDK to the target project.
If this is a new project or a Podfile does not exist, run the following command.
pod init
Step 2: Add the following line to the Podfile.
pod 'scg-messaing-ios'
Step 3: Install the pod using the following command.
pod install
After successful installation, the SCG messaging-ios SDK will be ready to use.
Interface
@interface SCGPush : NSObject + (instancetype _Nonnull) sharedInstance; @property (atomic, copy, nonnull) NSString* accessToken; @property (atomic, copy, nonnull) NSString* callbackURI; @property (atomic, copy, nonnull) NSString* appID; - (void) startWithAccessToken: (NSString* _Nonnull) accessToken appId: (NSString* _Nonnull) appId callbackUri: (NSString* _Nonnull) callbackUri; - (void) registerPushToken:( NSString* _Nonnull) pushToken withCompletionHandler:( void (^ _Nullable)(NSString * _Nullable token)) completionBlock failureBlock:( void (^ _Nullable)(NSError * _Nullable error)) failureBlock; - (void) unregisterPushToken:( NSString* _Nonnull) pushToken withCompletionHandler:( void (^ _Nullable)(NSString * _Nullable token)) completionBlock failureBlock:( void (^ _Nullable)(NSError * _Nullable error)) failureBlock; - (void) reportStatus: ( NSString* _Nonnull) messageId andMessageState: ( MessageState ) state completionBlock: ( void(^ _Nullable)(void) ) completionBlock failureBlock: ( void(^ _Nullable) (NSError* _Nullable error)) failureBlock; - (void) loadAttachmentWithMessageId:(NSString* _Nonnull) messageId andAttachmentId:(NSString* _Nonnull) attachmentId completionBlock:(void(^_Nullable)(NSURL* _Nonnull contentUrl, NSString* _Nonnull contentType))completionBlock failureBlock:(void(^_Nullable)(NSError* _Nullable error))failureBlock; - (void) resetBadgeForPushToken: (NSString* _Nonnull) pushToken completionBlock: (void(^_Nullable)(BOOL success, NSError* _Nullable error)) completionBlock; @end
How to use the SDK
- Import SDK into your source code
import SCGPushSDK
// Initialise instance of SDK
var
SCGPushSdk: SCGPush = SCGPush.sharedInstance()
- SCG Push SDK provides a singleton to access all the SDK methods across the application. To access singleton instance, use sharedInstance() accessor
|
- The sharedInstance will be available with a set of properties required for all operations, so before using SDK configure the properties
|
- To register your mobile application with the Omni-channel API you need to call next method (Example is shown for Firebase Cloud Messaging flow. For APN it will require APN type and APN token.)
|
- To unregister your mobile application with the Omni-channel API.
|
- To send a push notification state change callback event for the received notification.
|
- The push notification state needs to be updating prior to reporting the status for notification based on the predefined constants.
|
- To reset the badge count.
|
- To load attachments using the Omni-channel API.
|
Syniverse Android Mobile SDK for Push Notification.
The SDK is available via MavenCentral.
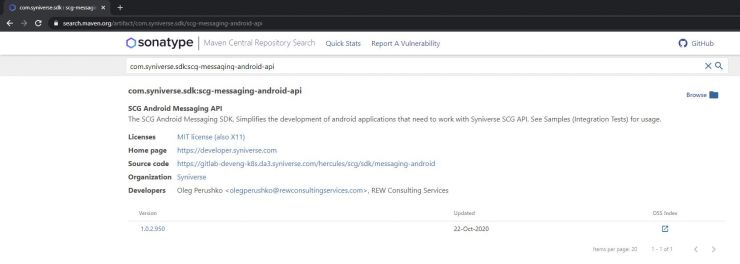
How to integrate the SDK into your mobile application
To integrate the SCG messaging-android SDK you need to add it to you project in dependencies tree
<
dependency
>
<
groupId
>com.syniverse.sdk</
groupId
>
<
artifactId
>scg-messaging-android-api</
artifactId
>
<
version
>1.0.2.950</
version
>
</
dependency
>
How to use the SDK
After successful installation SCG messaging-android SDK will be ready to use. Onwards will be explained an example how to manipulate SDK
- Public interface of the SDK with Session.class
|
- Import the SDK into your source code
|
- To create a new instance of the SDK
|
- To register your mobile application in Omni-channel API you need to call next method
|
- To unregister your mobile application with the Omni-Channel API
|
- To send callback for received message
|
Notification state needs to be updated prior to reporting status for notification based on the predefined constants.
|
- To load attachment with SCG API
|